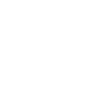
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs.
Curves in Games: The Art of Bezier Curves
Bezier curves are one of the most important concepts in computer graphics, known for their flexibility and precision in representing curves.
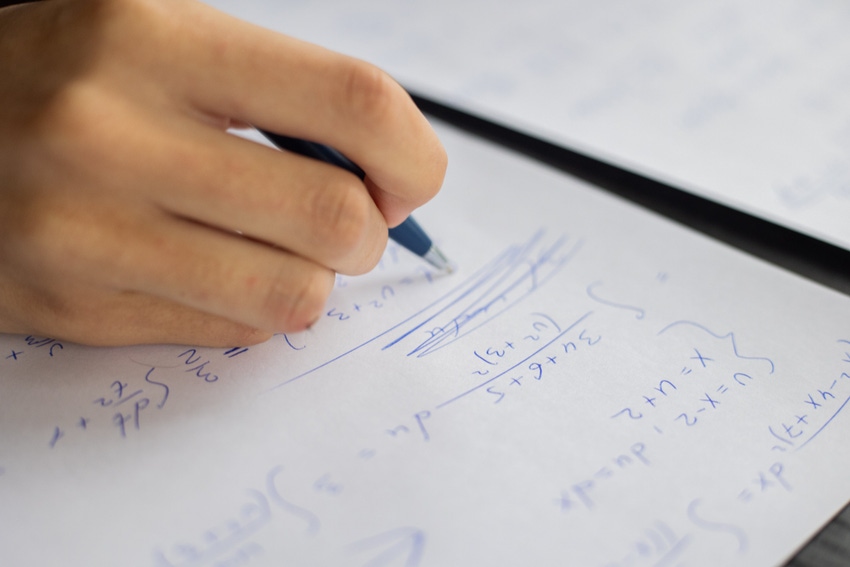
What is a Bezier curve?
Bezier curves are one of the most important concepts in computer graphics, known for their flexibility and precision in representing curves. The foundation of the mathematical principles of Bezier curves are the Bernstein polynomials which were established in 1912. There are countless usages of Bezier curves in the field of computer science, from animating smooth transitions for user interfaces and rendering dynamic fonts, to creating modeling tools.
In basic terms, Bezier curves are defined with a variable number of control points. With just two control points, it forms a straight line, also known as a linear Bezier curve. However, things get more interesting when there are more than two control points. A Bezier curve with three control points is a quadratic Bezier curve, while one with four points is a cubic Bezier curve, and so forth. Quadratic and cubic Bezier curves are particularly popular in tween animations because of their balance between computational simplicity and the capacity to represent an unlimited range of curves.
The parametric equation of a Bezier curve can be written as:
where:
B(t) is the point on the Bezier curve at the parameter t.
n is the degree of the Bezier curve.
P_i are the control points.
Implementation
In this section, we will explore the practical implementation of a Bezier curve using JavaScript. Starting with the binomial coefficient function. We can use a recursive approach due to its simplicity. However, recursion may not be the most efficient for large values due to the potential of stack overflow and excessive function calls. For those cases, an iterative solution would be more suitable. In the case of Bezier curves, the most commonly used variants are the quadratic and cubic ones, so a recursive implementation is enough:
Moving on to the core of our implementation which is the Bezier curve equation itself. To encapsulate our logic, we can create a class called ‘BezierCurve’ that has an array of control points. It also provides a method named ‘getPoint’ that returns a point on the curve with the t parameter as the input. The implementation is shown below:
With the ‘BezierCurve’ class in place, we now have a basic implementation of a parametric Bezier curve. In order to make things more interesting, below is a simple rendering of our ‘BezierCurve’ class in action:
The graph showcases a cubic Bezier curve, with its shape influenced by the four control points marked in red. In the next section, we will uncover some practical applications of the Bezier curves.
Use Bezier curves for animations
Bezier curves are commonly used in animation engines, offering a way to provide smooth transitions. For example, in web development, CSS provides native support for custom easing functions using Bezier curves. The same goes for software and game development, where UI design requires flexibility and modularity. As an example, a menu pop-up animation might need to start quickly and decelerate towards the end. Implementing such non-linear dynamic animation directly through code can be complex, and might have less control over variables in the animation.
With Bezier curves, this process can be simplified by defining a curve with four points, starting at (0, 0) and ending at (1, 1) to clamp the animation within a normalized range, and adding two control points to create the desired smooth effects. For the pop-up effect, the two middle control points can be at (0.25, 1) and (0.5, 1) respectively to generate a Bezier curve commonly known as “ease out quad” in CSS and tween engines.
To illustrate this, we will provide an example of how we can apply Bezier curves to a simple linear animation. Consider the following basic JavaScript code snippet that changes the transparency of a circle linearly:
The result animation looks like this:
To enrich this animation with a smoother transition, we can incorporate our ‘BezierCurve’ class by defining an “ease out quad” curve with control points of (0, 0), (0.25, 1), (0.5, 1) and (1, 1). Then replacing the linear alpha transition with the result of the ‘getPoint’ method, we can achieve a more refined animation:
The modification yields an animation that starts quickly and slows down gently.
Besides the common “ease out quad” ease type, there are numerous predefined ease types that can add more characteristics to animations. We can also easily create our own ease types with the help of websites such as https://easings.net/.
Use Bezier curves for movement
Apart from providing smooth animations for applications and games, Bezier curves can also be used to define a curved path for objects in games to move along. Consider a 2D shooter game where some enemies move in different paths. While straightforward paths like a straight line or even a circle can be hard-coded, this approach lacks flexibility and makes adjusting and visualizing the path challenging.
For this case, we can also use Bezier curves to both visualize and design complex paths with ease. Here’s an example of an object moving along a visualized curved path defined by a set of Bezier curve control points:
Here’s a basic idea of how it works: the Bezier curve provides a set of positions based on the t parameter which represents time. By updating the object’s position to correspond with these points, it’s able to traverse through the path smoothly.
Conclusion
That’s all for this post. There are still many other applications of Bezier curves. In conclusion, the flexibility and modularity of Bezier curves make it a powerful tool for developers. It simplifies complex curve equations into easier representations with control points.
References
https://easings.net/
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like